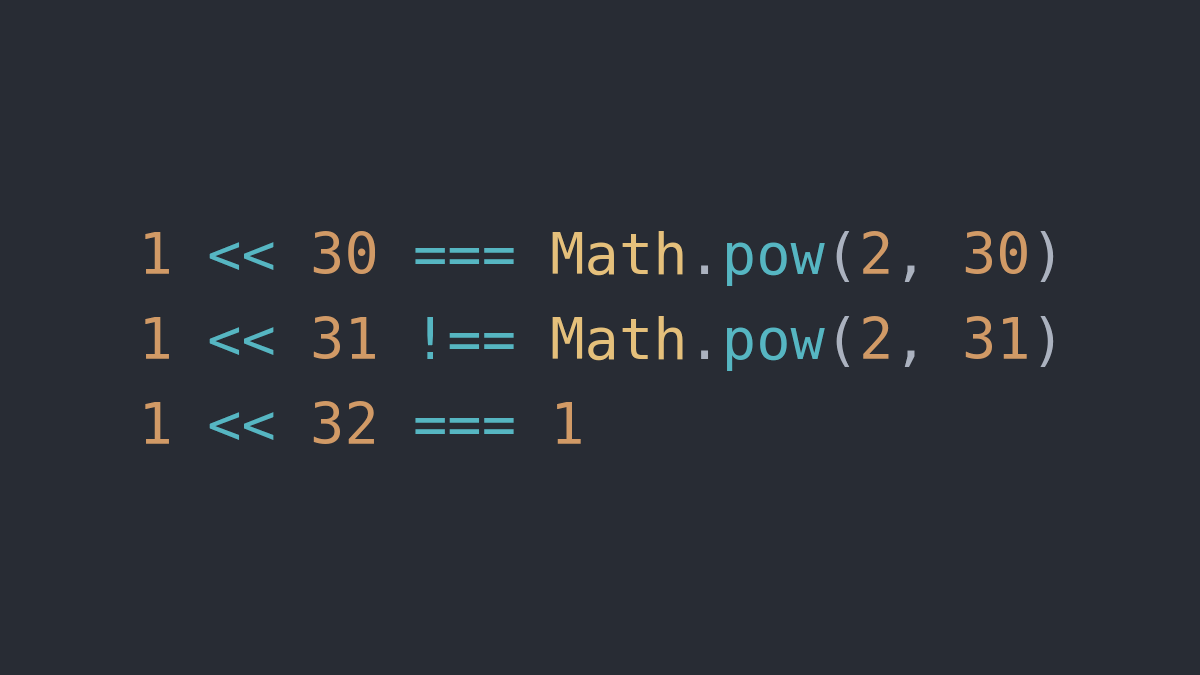
Bitwise left shift operator (<<
) is used instead of power of 2 (2n) operator on integer variable. This idiom may lead to strange result in JavaScript.
Bitwise operators in JavaScript converts their operands to signed 32-bit integer, not 64-bit integer (also known as long
in Java, long long
in C language).
Therefore, 1 << n
is not the same as Math.pow(2, n)
if n > 31
.
Not only the shift operator but also all the bitwise operators such as and(&
), or(|
), not(~
), XOR(^
) limited to process only 32-bit operands.
Solution
Mathematical operations
You would better to use Math.pow()
or **
(ECMAScript 2016) than <<
if you concern calculation.
Note that the safe integer range in JavaScript is -(253 - 1) inclusive to (253 - 1) inclusive since the Number
type uses IEEE-754 64-bit floating point number representation.
64-bit Long (long.js)
You may use long npm package if you concerned about only 64-bit wide integer.
Bitmap array
If you want to handle bit array with size > 64, you would better consider bitmap array, also known as bit vector.
There are several npm packages about bit array such as bitwise, bitset, fast-bitset, bit-vector. You may choose as you need.